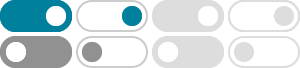
slice - How slicing in Python works - Stack Overflow
In Python 2.7. Slicing in Python [a:b:c] len = length of string, tuple or list c -- default is +1. The sign of c indicates forward or backward, absolute value of c indicates steps. Default is forward with step size 1. Positive means forward, negative means backward. a -- When c is positive or blank, default is 0. When c is negative, default is -1.
list - what does [::-1] mean in python - slicing? - Stack Overflow
Here is a simple cheat sheet for understanding Slicing, a[start:end] # items start through end-1 a[start:] # items start through the rest of the array a[:end] # items from the beginning through end-1 a[:] # a copy of the whole array a[start:end:step] # start through not past end, by step a[-1] # last item in the array a[-2:] # last two items in the array a[:-2] # everything except the last two ...
What is :: (double colon) in Python when subscripting sequences?
When slicing in Python the third parameter is the step. As others mentioned, see Extended Slices for a nice overview. With this knowledge, [::3] just means that you have not specified any start or end indices for your slice. Since you have specified a step, 3, this will take every third entry of something starting at the first index. For example:
How to slice a list in Python - Stack Overflow
2021年12月17日 · Slicing a python list. 0. Slicing a list specially. 1. Slice a list in python. 0. Slicing lists in python ...
How to slice a list from an element n to the end in python?
To my understanding, python slice means lst[start:end], and including start, excluding end. So how would i go about finding the "rest" of a list starting from an element n? Thanks a lot for all your help!
python - Big-O of list slicing - Stack Overflow
Say I have some Python list, my_list which contains N elements. Single elements may be indexed by using my_list[i_1] , where i_1 is the index of the desired element. However, Python lists may also be indexed my_list[i_1:i_2] where a "slice" of the list from i_1 to i_2 is desired.
Slicing a list using a variable, in Python - Stack Overflow
2012年5月13日 · Given a list a = range(10) You can slice it using statements such as a[1] a[2:4] However, I want to do this based on a variable set elsewhere in the code. I can easily do this for the first one...
reversing list using slicing [ : 0:-1] in Python - Stack Overflow
For the Python list slicing syntax, list[start:end:step] will get a sliced list with items starting with list[start], but list[end] is excluded. As a result, in your case, L[10:0:-1] will exclude L[0], i.e., 0, and L[10::-1] will work as you expect. When the start or end is a negative number, it means it counts from the end of the list.
Slicing 2D Python List - Stack Overflow
2022年4月5日 · Firstly note that python use indices starting at 0, i.e. for [1,2,3] there is 0th element, 1th element and 2nd element. [0:] means get list elements starting at 0th element, this will give you copy of list, [1:] means get list elements starting at 1th element, which will give you list with all but 0th element.
python - Does a slicing operation give me a deep or shallow copy ...
2016年8月3日 · The official Python docs say that using the slicing operator and assigning in Python makes a shallow copy of the sliced list. But when I write code for example: o = [1, 2, 4, 5] p = o[:] And when I write: id(o) id(p) I get different id's and also appending one one list does not reflect in the other list.